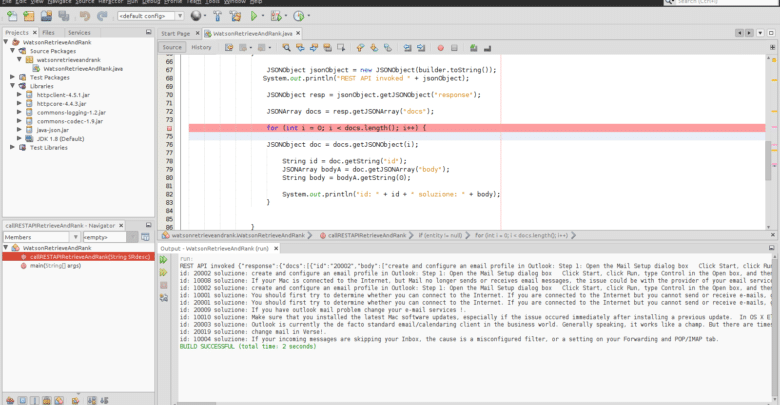
Developer
Simple REST client in Java for IBM Watson
As you know Maximo is currently based on a Java legacy infrastructure as long as IBM Watson is based on a cloud infrastructure with REST APIs. Here my proof of concept to invoke Watson by Control Desk.
To address my requirement without use the current and cool JavaScript frameworks I developed a simple REST client in Java plus a bunch of jar libraries. I hoping that it will help someone in some way.
To invoke the IBM Watson REST APIs regarding the Retrieve and Rank service:
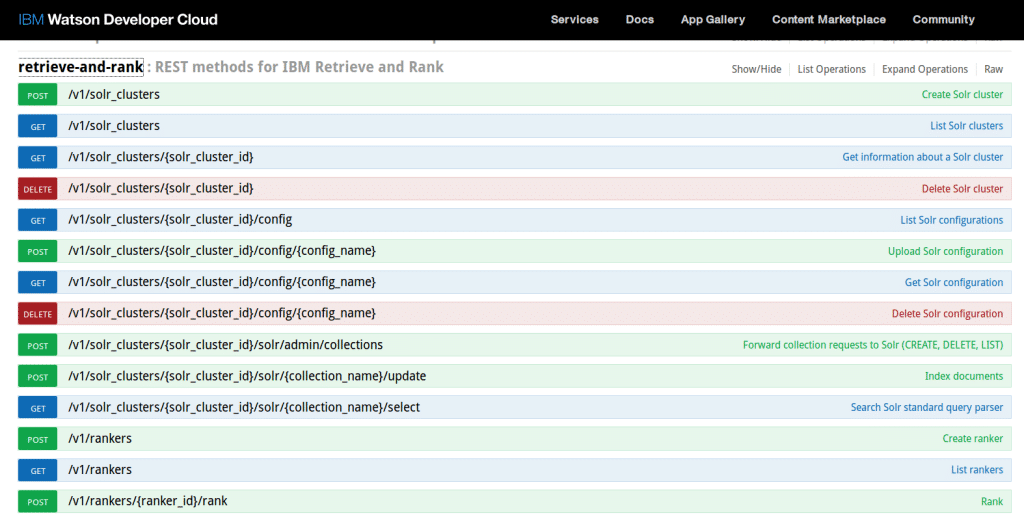
I developed the following Simple REST client in Java
/* This program is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. */ package watsonretrieveandrank; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.client.ClientProtocolException; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpPost; import org.apache.http.entity.StringEntity; import org.apache.http.impl.client.DefaultHttpClient; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; /** * * @author Mario Noioso */ public class WatsonRetrieveAndRank { public static void main(String[] args) throws Exception { callRESTAPIRetrieveAndRank("mail configuration problem"); } public static void callRESTAPIRetrieveAndRank(String SRdesc) throws ClientProtocolException, IOException, JSONException { @SuppressWarnings("deprecation") HttpClient client = new DefaultHttpClient(); StringBuilder builder = new StringBuilder(); String url = "https://c0076286-3009-40c2-886f-bf6d833XXX:[email protected]/retrieve-and-rank/api/v1/solr_clusters/sce5325126_b64b_4141_af68_31af26cf7683/solr/example-collection/select?q="+ SRdesc + "&wt=json&fl=id,body"; url = url.replaceAll(" ", "%20"); HttpPost post = new HttpPost(url); post.setHeader("Accept", "application/json"); post.setHeader("Content-type", "application/json"); StringEntity input = new StringEntity(""); post.setEntity(input); HttpResponse response = client.execute(post); HttpEntity entity = response.getEntity(); if (entity != null) { InputStream inputStream = entity.getContent(); BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream)); for (String line = null; (line = bufferedReader.readLine()) != null;) { builder.append(line).append("\n"); } JSONObject jsonObject = new JSONObject(builder.toString()); System.out.println("REST API invoked " + jsonObject); JSONObject resp = jsonObject.getJSONObject("response"); JSONArray docs = resp.getJSONArray("docs"); for (int i = 0; i < docs.length(); i++) { JSONObject doc = docs.getJSONObject(i); String id = doc.getString("id"); JSONArray bodyA = doc.getJSONArray("body"); String body = bodyA.getString(0); System.out.println("id: " + id + " solution: " + body); } } } }
Some details:
- Invoking the search Solr standard query api
- Using the following Jars: httpclient-4.5.1.jar , httpcore-4.4.3.jar , commons-logging-1.2.jar , commons-codec-1.9.jar , java-json.jar
- Using the JSONObject to handling the HTTP response with JSON content
Here the output from my simple REST client in Java for IBM Watson

Your comments and suggestions are welcome.
Hi Mario,
I`m doing an application using retrieve and rank and now I need to save a json in the cluster, I tried to use this code
String url = “https://XXXXXX.com/LogFinderGateway/LogFinder?SRdesc=”;
HttpPost request = new HttpPost(url);
StringEntity params =new StringEntity(“details={\”id\”:\”7777777777\”,\”body\”:\”bla12345\”} “);
request.addHeader(“content-type”, “application/json”);
request.addHeader(“Accept”,”application/json”);
request.setEntity(params);
HttpResponse response = httpClient.execute(request);
System.out.println(response.toString());
I receive the result
HTTP/1.1 200 OK [Date: Wed, 28 Jun 2017 21:19:16 GMT, Server: Apache, Content-Length: 595, Connection: close, Content-Type: text/html;charset=ISO-8859-1] org.apache.http.conn.BasicManagedEntity@58a90037
But it was not persisted.
Do you have any ideas? I tried to find an example that persist data in cluster using R&R and found nothing.
Thanks a lot in advance
Best Regards
Lazara
very useful ! thanks for sharing